- #javaScript
- #arrays
- #snippets
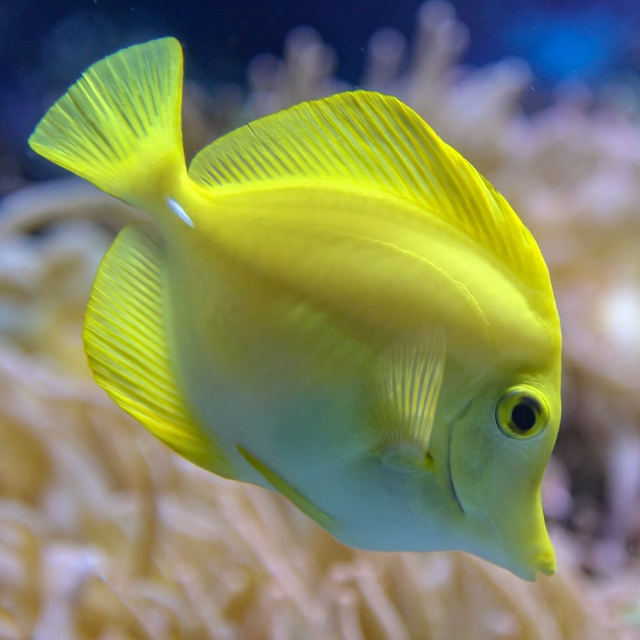
Introduction
All web applications draw on data to make the app work and arrays are most likely used for storing that data. It is important therefore for developers to know how to add data to arrays.
Add an element to the end of an array
Take a simple of string elements.
const demoArray = ['Elsie', 'Joe', 'Sarah']
We could use the array length property to add an element to the end of the array.
demoArray[demoArray.length] = 'Jane';
// ['Elsie', 'Joe', 'Sarah', 'Jane']```
But this is a bit long winded and instead the built in method push( ) can be used.
demoArray.push('John')
// ['Elsie', 'Joe', 'Sarah', 'Jane', 'John']
Add an element to the beginning of an array
If we know that an array is empty we can add the first element by using the 0 index.
const anArray = []
anArray[0] = 'Hello world'
// ['Hello world']
However if we do that again we do not add an element but overwrite the first element in the array.
const anArray = []
anArray[0] = 'Help the world'
// ['Help the world']
The best way to add an element to the beginning of an array is by using the built in unshift( ) method.
demoArray.unshift('Erin')
// ['Erin', 'Elsie', 'Joe', 'Sarah', 'Jane']
Remove element from the end of an array
The opposite of ‘push’ is the pop( ) method. Using this built in method we can pop an element off the end, like so:
demoArray.pop()
// ['Erin', 'Elsie', 'Joe', 'Sarah', 'Jane']
Remove element from beginning of an array
The opposite of ‘unshift’ is the shift( ) method. Using this built in method we can shift an element from the beginning, like so:
demoArray.shift()
// ['Elsie', 'Joe', 'Sarah', 'Jane']
Summary
In summary to add elements to an array use push to the end and unshift to the beginning. To remove elements use pop from the end and shift from the beginning.
That’s it.
Thank you for stumbling across this website and for reading my blog post entitled How to add elements to and remove elements from an array