- #javaScript
- #arrays
- #snippets
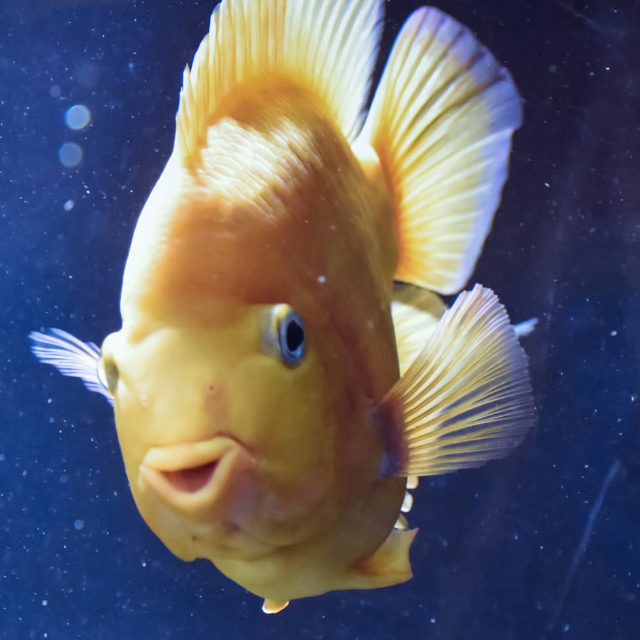
Ways of calculating tax of prices in an array
Let us create an array holding different prices of items in a shop.
const prices = [30, 49, 22, 11, 10, 19];
The tax percentage to be used is 17.5%.
const tax = 17.5;
Using .map()
Each item needs tax added to them. Using the map() method will create a new array with the results of the calculation function written inside the map parentheses.
let prices_plus_tax = prices.map(price => price + (price / 100 * tax))
This results in the following prices:
[35.25, 57.575, 25.85, 12.925, 11.75, 22.325]
But there is a problem, can you see what needs fixing? Shops display items with two decimal places, not three. We can adjust our map() method calculation to deal with this.
Fixing decimal places
In the code below parentheses have been placed around the calculation and the number prototype toFixed() has been applied to the whole calculation. The number 2 is added within the toFixed(2) parentheses to ensure that the new array contains numbers with two decimal places.
prices_plus_tax = prices.map(price => (price + (price / 100 * tax)).toFixed(2))
The calculation results now have 2 decimal places, but notice that each element has been turned into a string instead of a number!
['35.25', '57.58', '25.85', '12.93', '11.75', '22.32']
Converting the strings to numbers
To fix this add a + before the whole calculation
prices_plus_tax = prices.map(price => +(price + (price / 100 * tax)).toFixed(2))
Now in the console we have the required result.
[35.25, 57.58, 25.85, 12.93, 11.75, 22.32]
Tadaa!
Thank you for stumbling across this website and for reading my blog post entitled Add a tax percentage to array of prices