- #snippets
- #css
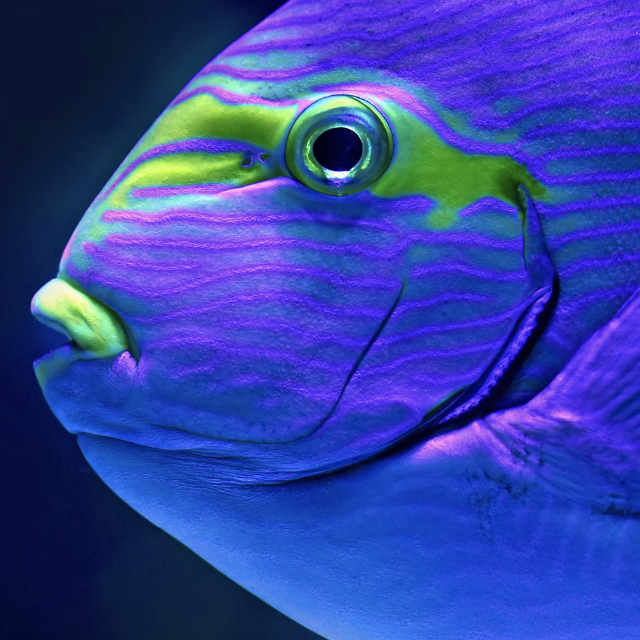
Introduction
Repsonsive web design has been with us for over a decade. Flexbox, CSS Grid and Media queries provide web developers the tools to style applications across browsers on all devices. There is a new kid on the block: CSS container gives us the ability to control responsivenesss at a micro-level.
Media query or CSS container?
The media query option works on break points that are associated with browser widths. For example, I often use 576 and 768 pixels that are common for browsers on phones and tablets.
The CSS container option allows extra control, not based on the browser width but on the width of components on the application. Let’s take a look.
Example project
I am creating a game and want a row of cells to fill the screen on a small device (phone). On a larger screen the cells can be 50 pixels width and height. The HTML below adds 8 div elements with a class of cell to a container element with a class of board.
<div class="board">
<div class="cell"></div>
<div class="cell"></div>
<div class="cell"></div>
<div class="cell"></div>
<div class="cell"></div>
<div class="cell"></div>
<div class="cell"></div>
<div class="cell"></div>
</div>
Nothing special so far. Here is the CSS.
.cell {
width: 50px;
height: 50px;
background-color: rebeccapurple;
border-radius: 50%;
margin: 5px;
}
Again, nothing special here. Now we add the interesting CSS container code to the parent div with the class board. The container-type is required and is set to inline-size. The container-name is optional but I think pretty useful as it adds defined control for larger applications.
.board {
display: flex;
justify-content: center;
border: 1px solid red;
/* css container! */
container-type: inline-size;
container-name: board;
}
Using the @ symbol like a media query, we can add @container.
@container board (max-width: 501px) {
.cell {
/* add styling */
}
}
Notice the container-name has been used and a max-width. When the container width is less than 501x, the internal styling will be applied.
Style cells for small screens
To dynamically apply a width and height to the cell class without using JavaScript, I add a —fullWidth variable to the CSS body using viewport width (vw).
body {
--fullWidth: 100vw;
}
The CSS calc option is applied to divide the device viewport width by ten and apply it to the cell width and height.
@container board (max-width: 501px) {
.cell {
width: calc(var(--fullWidth) / 10);
height: calc(var(--fullWidth) / 10);
}
}
Summary
The CSS container property was introduced in 2023 and has wide browser support. It allows micro-styling on components, negating the use of complex media queries with chained classes and sub-classes.
Thank you for stumbling across this website and for reading my blog post entitled The CSS container property.