- #javaScript
- #arrays
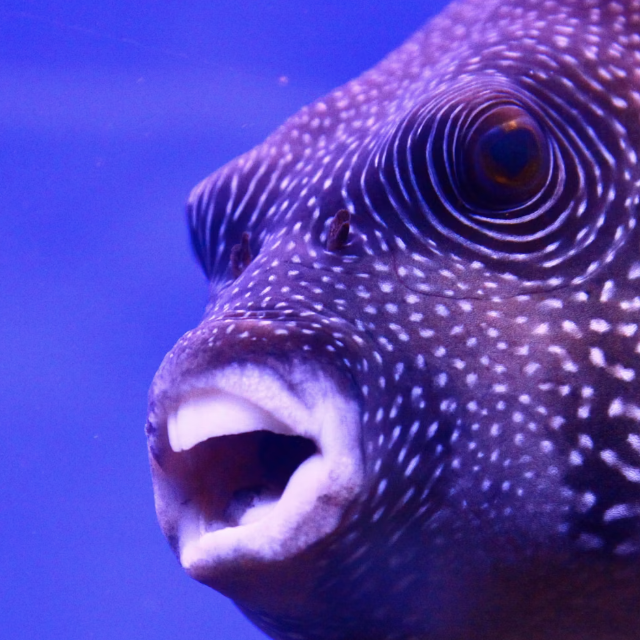
Introduction to arrays
I first published Arrays in JavaScript post on my website but needed to replicate it here. Arrays are so important in JavaScript as they hold data for most web applications and websites.
What is an array?
Arrays are used to store collections of related values that can be manipulated in an application. An array is stored as a named variable, preferably with a name that makes sense as this will make your code more readable.
Each value within an array is called an element. The elements can be any datatype: string, number, boolean, null, undefined and can even be an object, an array or an empty element! Let us consider what arrays could be used for.
Use cases of arrays
When learning JavaScript it is initially tricky to understand how arrays can be used. Right now you are reading a blog post that is an element contained in an array of posts.
If I chose to have subscribers on this website, I would most likely create an array of subscribers! If a user was not in the array, they would need to subscribe.
A restaurant app might have an array of meals to be displayed to customers. The same array could include recipes to display in the kitchen. The same array could be used by the front-of-house staff for taking customer orders.
An airport app might use array(s) for each airline’s departure and arrival details. A complex array combining all airline companies could be used to display travel information on arrival and departure boards. A similar array use could be used for a railway network too!
A banking app would likely use complex array(s) to store customer information such as account details, login info, income and outgoings etcetera.
Having provided a few ideas on what arrays could be used for, let us explore how to create arrays and add data to them.
Creating an array
A beautiful (but sometimes confusing) aspect about JavaScript is that there are often different ways to achieve the same result. Here is a list of four ways to create an array:
- Array literal (the simplest way)
- Array constructor
- Array.of() (introduced in ES6)
- Array.from() (introduced in ES6)
Array literals
Create a named variable and add square brackets [ ], adding the elements within the brackets. If you add strings, they must be within “inverted commas”. Numbers do not need inverted commas, like so:
const myFirstArray = [1, 2, 3];
Array constructor
Create a new Array( ) adding elements within the parentheses, like so:
const wild_animals = new Array('lion', 'tiger', 'ape');
// ['lion', 'tiger', 'ape']
Array.of( )
Create an array with the ES6 Array.of( ) function, adding the elements within the parentheses, like so:
const farm_animals = Array.of('cow', 'goat', 'sheep');
// ['cow', 'goat', 'sheep']
Array.from( )
Copy an array with the ES6 Array.from( ) function, passing the original array name into the parentheses.
const copiedFirst = Array.from(myFirstArray);
// [1, 2, 3]
Spread operator
There is another way to copy data from one array to another, that is using the spread operator. This uses 3 full-stops then the original array name without a space. Let us copy the farm and wild animal arrays into a new array, for example:
const all_animals = [...farm_animals, ...wild_animals];
// ['cow', 'goat', 'sheep', 'lion', 'tiger', 'ape']
Accessing array data
We now know that we can store data in arrays and have seen a few ways how to create an array but how can we access, or read, the data? The array property length and its built-in indexing are used for this, let us take a look.
Array length
Every array has a property called length and this can be useful in programming. The length of an array is determined by the number of elements within it. An empty array has a length of 0 (zero). The all animals array above has a length of 6. We can determine the length property by arrayName.length, like so:
farm_animals.length; // 3
all_animals.length; // 6
So what?
There may be a time when you need a conditional statement to check whether an array is empty or not, for example:
const subscribers = [];
if (subscribers.length === 0) {
console.log('There are no subscribers yet');
}
Array index
Every element in an array has it’s own index number. It is important to remember that this index starts from 0 (zero). Therefore the first element in an array has an index of 0 (zero), the second element has an index of 1, and so on. To access the data in the array we use the square-bracket notation [ ], adding the index number inside, like so:
all_animals[0]; // cow
all_animals[1]; // goat
all_animals[4]; // tiger
If an element does not exist in the array undefined will be returned.
const emptyArray = [];
emptyArray[2]; // undefined
all_animals[7]; // undefined
Thinking about length and index
The length is based on the number of elements in the array but the index system starts at zero. So there is a discrepancy. If you want to access the last element in the array, we could use length - 1 as the index, like this:
const users = ['Erin', 'Elsie'];
users[users.length - 1];
// Elsie
Changing, deleting and adding elements in an array
So far we discovered four ways of creating arrays and how to store and access array elements. Now we can take a look at how to change element values.
To change the value of an element in array use the array name and [ ] with no spaces, an equals sign (=) then the new value. The new value overwrites the old value, as seen in the example below.
const wild_animals = new Array('lion', 'tiger', 'ape');
// ['lion', 'tiger', 'ape']
wild_animals[0] = 'Elephant'
// ['Elephant', 'tiger', 'ape']
In the next example is a game of TicTacToe, OXO, or Noughts and Crosses depending where you are in the world. Create an initial array of 9 empty elements using the new Array() function.
If the first player places an X in the 6th position, an ‘x’ is added to the 5th element of the array. The next player clicks on the 4th position and an ‘o’ updates the 3rd element of the array. This continues until there is a winner or stalemate (we’ll figure out how to identify winner in a seperate blog post!).
In the meantime study the syntax below on how to change the value of an element in an array.
const tictactoe = new Array(9);
// [empty × 9]
tictactoe[5] = 'x';
// [empty × 5, 'x', empty × 3]
tictactoe[3] = 'o';
// [empty × 3, 'o', empty, 'x', empty × 3]
Emptying an array
There are two ways to remove all elements from an array. First we could make use of the length property by stating that the length is zero.
const numbers = [3, 6, 9, 12, 15];
// [3, 6, 9, 12, 15]
numbers.length = 0;
// [ ]
The second way is to declare the variable as an empty array.
const numbers = [3, 6, 9, 12, 15];
// [3, 6, 9, 12, 15]
numbers = [ ];
// [ ]
Add elements to an array
To add elements to an array built in methods can be used. The push( ) method adds an element to the end of the array and the unshift( ) methods adds an element to the beginning, like so:
const birds = ['Waders'];
// ['Waders']
birds.push('Owls');
// ['Waders', 'Owls']
birds.unshift('Falcons');
// ['Falcons', 'Waders', 'Owls']
Remove elements from an array
The built in methods pop( ) and shift( ) remove the last and first elements respectively. Take a look:
// ['Falcons', 'Waders', 'Owls']
birds.pop();
// ['Falcons', 'Waders']
birds.shift();
// ['Waders']
More complex arrays
So far we have dealt with simple arrays but they can be more complex. When creating an application, I would advise you to carefully consider how you will stucture your data before typing any code. Doing so will avoid heartache later :)
Array of objects
I will write a blog on using objects for holding data in due course but at this point it is worth understanding that you can add objects as elements in an array. This blog post uses such a data structure.
const allBlogs = [
{
title: 'Arrays in JavaScript',
author: 'Mike',
tags: ['array', 'JavaScript', 'learning in public', 'programming'],
},
{
title: 'Array methods in JavaScript',
author: 'Mike',
tags: ['array', 'methods', 'JavaScript'],
},
];
To access the title of the second array, we would use the square brackets as before, then use the dot notation then the title key in the object, like this:
allBlogs[1].title;
// Arrays methods in JavaScript
Nested arrays
Arrays can be added as elements in an array too. If used, these are called nested array(s). Here is an example of a nested array:
const users = [
['George', 'Chris', 'Mike'],
['Naomi', 'Fiona', 'Sue'],
];
Don’t panic! The nested arrays all have a length property and their own index too.
users[0][2]; // Mike
users[0][0]; // George
users[1][0]; // Naomi
Summary of JavaScript array notes
This article described:
- what arrays are and possible use cases.
- four ways of creating arrays including array literals, array constructor, array.of and array.from.
- how to manually add data to an array.
- how to access elements in an array.
- how to change array element values.
- how to add and remove data using built in methods.
- more complex arrays with objects and nesting.
What next?
JavaScript arrays can be further manipulated using a range of built-in array methods. They can also be iterated, or looped over, to enhance the use of array data. Loops and methods are subjects of my JavaScript notes.
Thank you for stumbling across this website and for reading my blog post entitled Arrays in JavaScript