- #objects
- #javaScript
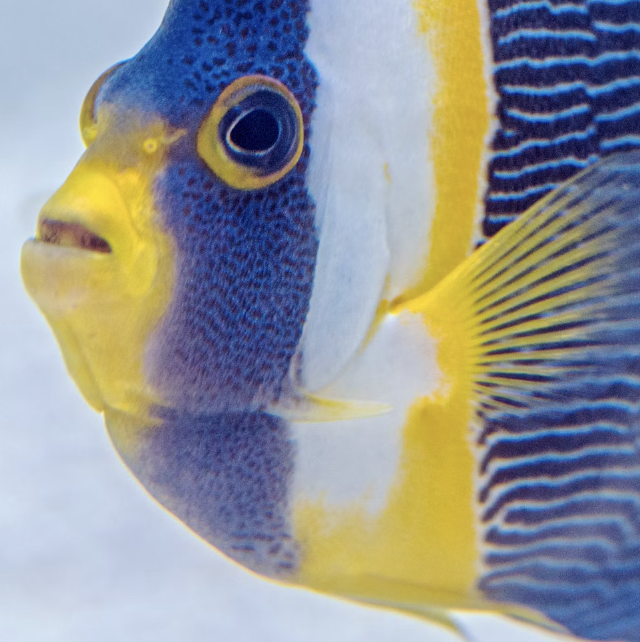
What is an Object?
Objects are used for storing and manipulating data but in a more eye-pleasing manner than arrays. An Object is stored as a named variable, preferably with a name that makes sense as this will help make your code more readable. The data within the object is held in key: value pairs called properties.
Property values can be any datatype: string, number, boolean, null, undefined, an array, another Object or a function (called a method).
Use cases
A case that I use a lot when programming a web app is to place DOM variables within an Object, so they are unavailable in the Global scope. Here is such an variable named ui (short for user interface):
const ui = {
logInBtn: document.getElementById('login');
logOutBtn: document.getElementById('logout');
}
I would then, for example, add an eventListener to each using the following syntax.
ui.logInBtn.addEventListener('click', logUserIn);
ui.logOutBtn.addEventListener('click', logUserOut);
Another use case is one of my objects created when debeloping my take on the classic Tetirs game. You can see here that the property values include numbers, arrays and booleans.
const data = {
origin: 4,
twist: 0,
game: [],
colrs: ['crimson', 'dodgerblue', 'rebeccapurple', '#009432', '#ff793f', 'teal'],
shapeArr: [0, 3],
inPlay: false,
shapeOnRight: false,
shapeOnLeft: false,
atBase: false,
level: 0,
speed: 300,
score: 0,
highscore: 0,
gameover: false
}
Creating an Object
A beautiful (but sometimes confusing) aspect about JavaScript is that there are often different ways to achieve the same result. Here are 3 ways to create an Object:
- Object literals
- Object constructor use the new keyord
- Object.create()
Object literals
The syntax uses curly braces in which properties are comma seperated. A property is defined using a colon : seperator between the key and the value.
const passenger = {
first_name: 'Naomi',
last_name: 'Smith',
passport: '9876432G',
};
If a comma is missing between any of the properties a syntax error is thrown.
Uncaught SyntaxError: Unexpected identifier ' '
Object constructor
The new keyword creates and initialises a new empty object.
const myWebsite = new Object();
// {}
It is worth noting that you cannot create objects of the same name but keys of the same name can be used in different objects. In the event of mistakenly creating an object of the same name the following error is thrown:
Uncaught SyntaxError:
Identifier ' ' has already been declared
Object create()
Using the Object.create() method adds the key value pairs to the object prototype. We will take a look at prototypes below.
Accessing and manipulating object data
To access property values from an object we use the dot notation or square bracket operators.
Dot notation
Using the dot notation is the cleanest and easiest to read. We call on the object variable then a dot then the key of the value required.
const dev = {
firstname: 'Mike',
};
// dot notation
dev.firstname;
// 'Mike'
Brackets notation
If using the square bracket operators [ ], the key must be passed in as a string, like so:
// square brackets
dev['firstname'];
// 'Mike'
If the string is not used the following error will occur.
// square brackets
dev[firstname];
// error message
Uncaught ReferenceError: firstname is not defined
Adding data to an object
After an object has been created more properties can be added
dev.lastname = 'Smith';
In calling the dev variable, we see the lastname property has been added.
dev;
// {firstname: 'Mike', lastname: 'Smith'}
Changing the value of a object property
Updating or changing the value is simple. Call the variable name, dot, key and equals, than the new value. We can also use the square bracket but don’t forget the string!
// dot notation
dev.firstname = 'Naomi';
// {firstname: 'Naomi', lastname: 'Smith'}
// bracket notation
dev['lastname'] = 'Toft';
// {firstname: 'Naomi', lastname: 'Toft'}
Deleting an object property
Simply use JavaScript’s delete operator followed by the variable name dot key
delete dev.lastname;
// {firstname: 'Naomi'}
Functions in objects
In addition to key value pairs, we can add functionality that is locally scoped within the object to make use of its data.
const me = {
first: 'Mike',
last: 'Toft',
info() {
return `${this.first} ${this.last} is a JavaScript developer`;
},
};
me.info();
// Mike Toft is a JavaScript developer
Note the use of the keyword this. It points to object itself and can therefore call upon the internal keys. If this is omitted an error message is thrown:
// no this in the return statement
info() {
return `${first} ${last} is a JavaScript developer`
}
// Uncaught ReferenceError: first is not defined at Object.info
The function above could be destructured and it will output the same result.
const me = {
first: 'Mike',
last: 'Toft',
info() {
const { first, last } = this;
return `${first} ${last} is a JavaScript developer`;
},
};
document.getElementById('job').textContent = me.info();
The next example is an object with two functions that use no data from within the object.
const sums = {
add: function (x, y) {
return x + y;
},
subtract: function (x, y) {
return x - y;
},
};
These functions are called like this:
sums.add(10, 11);
// 21
sums.subtract(50, 11);
// 39
Of course, we could simplify the code with arrow functions.
const maths = {
multiply: (x, y) => x * y,
divide: (x, y) => x / y,
};
maths.multiply(3, 8);
// 24
maths.divide(12, 4);
// 3
Objects as reference types
In JavaScript, objects are called reference types because they are not stored directly in variables. Instead, variables store a reference to the object. This means that when you assign an object to a variable, you are not actually copying the object, just the reference to it. Let us have a look.
First create an object with object literals (curly braces). Then, just for practice, create an object with the new keyword, passing in the first object as an argument.
const learn = { code: 'CSS' };
const learning = new Object(learn);
Both variables reference the same object. See what happens when the learn.code value is changed:
learn.code = 'JavaScript';
console.log(learn, learning);
// {code: 'JavaScript'}, {code: 'JavaScript'}
They are both the same because both variables reference the same object the values are exactly the same.
The spread operator
The referencing behaviour above does not work if the spread operator (three dots) is used. This is because the spread operator does not copy the reference, instead it loops the original object properties and adds the key value pairs to the new object. Let’s take a look ..
const obj_1 = { code: 'CSS' };
const obj_2 = { ...obj_1 };
// obj_1 {code: 'CSS'}
// obj_2 {code: 'CSS'}
obj_1.code = 'JavaScript';
// obj_1 {code: 'JavaScript'}
// obj_2 {code: 'CSS'}
See the difference, now if a value changes in the original object it will not be reflected in the new object.
Object protoypes
Every object created in JavaScript has an Object.prototype It has a number of built-in properties and methods, such as the toString() method and the hasOwnProperty() method.
When we create a new object with the Object.create() function, the key value pairs are passed to another Object.prototype. These prototypes form what is known as a prototype chain.
When we want to retrieve a value, if the property is not found on the object, JavaScript will look for it on the prototype. If not found there the process continues up the prototype chain until the root Object.prototype is reached. If the property does not exist undefined will be returned.
const x = { a: 1, b: 2 };
const y = Object.create(x);
y.b;
// 2
y.c;
// undefined
Thank you for stumbling across this website and for reading my blog post entitled Objects in JavaScript