- #javaScript
- #snippets
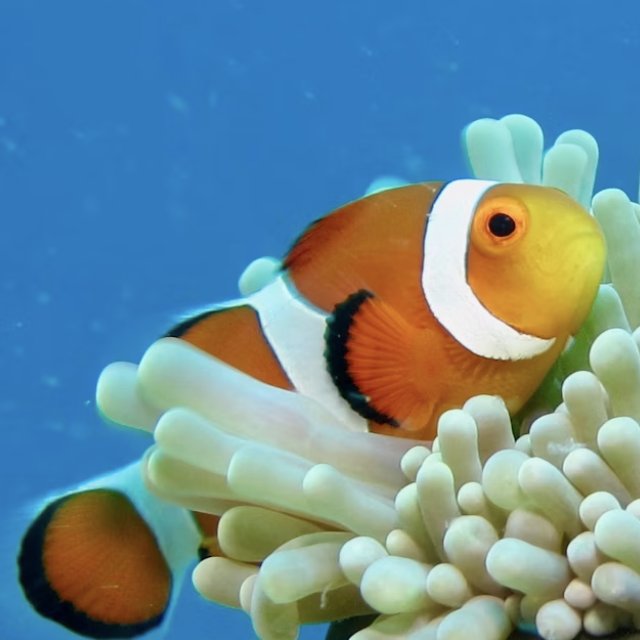
The problem to solve is how to round an array of decimal numbers up to whole integers. The numbers variable points to such an array.
const numbers = [1.2, 2.49, 3.5, 5.7]
The Math.round() method should solve the problem. Let’s see.
let rounded = []
numbers.forEach(n => rounded.push(Math.round(n)))
// rounded [1, 2, 4, 6]
The result is not quite what we want. The decimals below .5 are rounded down to the nearest integer whereas the decimals above .5 are rounded up.
Instead let’s try the Math.ceil() method.
let ceiled = []
numbers.forEach(n => ceiled.push(Math.ceil(n)))
// ceiled [2, 3, 4, 6]
This works a treat. Therefore, to round a decimal number up to a whole integer use the Math.ceil() method.
Thank you for stumbling across this website and for reading my blog post entitled Round a decimal number up with JavaScript