- #javaScript
- #arrays
- #snippets
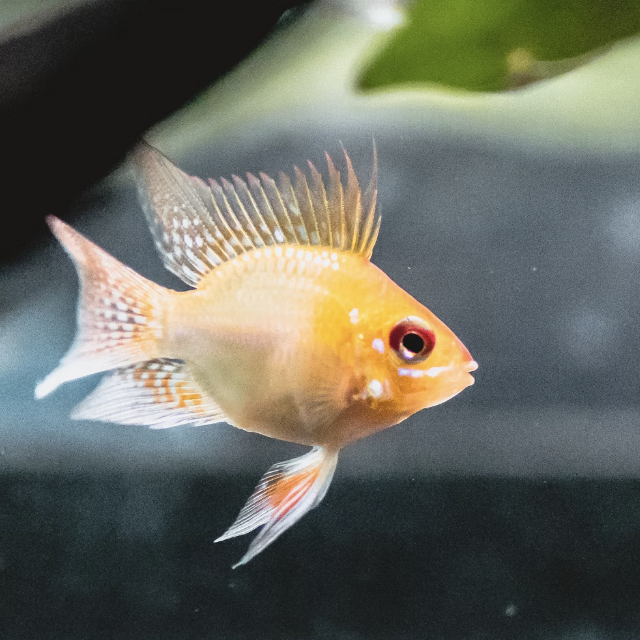
Shuffling an array of items with JavaScript
Now and again you might need to shuffle the items in an array. For example if developing a game that uses a deck of cards, or perhaps the Suduko game.
The Fisher-Yates Shuffle Algorithm can solve our problem. Here is the explanation on Wikipedia: Fisher-Yates Shuffle. It works whether the array contains numbers or strings. Let’s take a look.
Here is an array of numbers.
const numbers = [1,2,3,4,5,6,7,8,9]
And here is an array of strings.
const strings = ['one', 'two', 'three', 'four']
Notice that the shuffle function iterates over the array (arr) backwards, using i - - The j variable creates a random integer between zero and the length of the array. Finally, the array items at i and j swap positions.
function shuffle(arr) {
for (let i = arr.length - 1; i > 0; i--) {
const j = Math.floor(Math.random() * arr.length);
[arr[i], arr[j]] = [arr[j], arr[i]];
}
return arr;
}
Now call the function passing in the numbers array as the argument.
const shuffledNumbers = shuffle(numbers);
console.log(shuffledNumbers)
// [4, 1, 2, 3, 8, 7, 6, 5, 9]
If you copy the code on here, you will get a different shuffle result because the chance of getting the same random numbers is near impossible.
Now call the function passing in the strings array as the argument.
const shuffledStrings = shuffle(strings)
console.log(shuffledStrings)
// ['three', 'one', 'four', 'two']
Again, if you copy the code here, you will get a different shuffle result.
That’s all for now.
Thank you for stumbling across this website and for reading my blog post entitled Shuffle array items with JavaScript.