- #javaScript
- #arrays
- #snippets
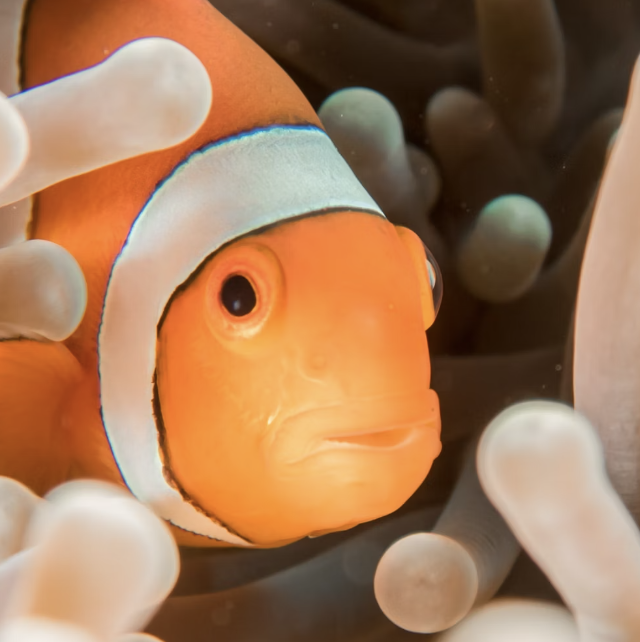
Sorting an array of numbers with JavaScript
Here is an array of numbers jumbled in a random order.
const jumbled_numbers = [7, 3, 46, 5, 11, 2, 6, 23, 4, 1]
If we apply the .sort() method to the array, we get a curious result.
jumbled_numbers.sort();
// [1, 11, 2, 23, 3, 4, 46, 5, 6, 7]
Sort numbers in ascending order
Now create a variable ‘ascending_numbers’ and apply the sort() method to the original array. But this time we compare each array element (a) with its neighbours (b).
const ascending_numbers = jumbled_numbers.sort((a, b) => a - b)
Logging ‘ascending_numbers’ to the console, you will see:
[1, 2, 3, 4, 5, 6, 7, 11, 23, 46]
Sort numbers in descending order
Create a variable ‘descending_numbers’ and apply the sort() method to the original array.
const descending_numbers = jumbled_numbers.sort((a, b) => b - a)
Logging ‘descending_numbers’ to the console, you will see:
[46, 23, 11, 7, 6, 5, 4, 3, 2, 1]
Find the highest number in an array
Armed with out knowledge we can now find the highest number in an array.
jumbled_numbers.sort((a, b) => a - b);
// [1, 2, 3, 4, 5, 6, 7, 11, 23, 46]
const highest = jumbled_numbers[jumbled_numbers.length - 1];
// 46
Find the lowest number in an array
Having sorted the array in ascending numerical order, the lowest number in the array will always be at index 0.
const lowest = jumbled_numbers[0];
// 1
Thank you for stumbling across this website and for reading my blog post entitled JS Arrays in numerical order