- #snippets
- #javaScript
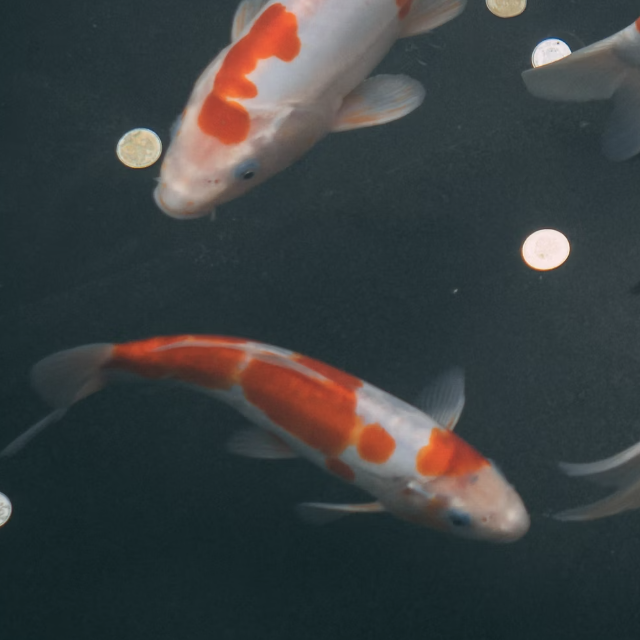
Introduction
Let’s say we want to input a number representing a total number of minutes and then calculate the hours and minutes and display them in the browser. For example the total number of 384 minutes would be 6 hours 24 minutes and 1131 minutes would result in 18 hours 51 minutes.
HTML setup
Within the body tag of the HTML file, simply add a number input and a paragraph tag to display the result. This p tag is given an id ‘result’.
<input type="number">
<p id="result"></p>
JavaScript event listener
In the JavaScript add an eventListener on the input field. Because it is the only input field in the HTML querySelector(‘input’) can be used, otherwise a unique id would be required.
document.querySelector('input').addEventListener('input', displayHHMM);
The app is now listening for any user input in the field. When an input is made the displayHHMM function is called.
If change is used instead of input the function will be called when the user moves off the input field.
The JavaScript function
Initially an arrow function is used to capture the user input event. The variable input records the value of the HTML input field.
const displayHHMM = (e) => {
let input = (e.target.value);
}
The hours can be calculated by dividing the input number by 60. Minutes remaining is calculated with modulo % feature. This calculates the remainder of the division, for example 62 divided by 60 has a remainder of 2. Notice below the .toFixed() method is used to deal with any decimal numbers from the user input. The app is calculatin minutes not seconds.
const displayHHMM = (e) => {
let input = (e.target.value);
let hh = Math.floor(input / 60);
let mm = (input % 60).toFixed()
}
Displaying the result
To tweak the textual result a ternary operator is used to cater for a single hour or mutliple hours. A regular if statement could be used but the ternary is a bit cleaner in this case.
The hours variable will either record ‘1 hour’ or ‘n hours’. Additionally, the minutes variable will either record ‘1 minute’ or ‘n minutes’.
const displayHHMM = (e) => {
let input = (e.target.value);
let hh = Math.floor(input / 60);
let mm = (input % 60).toFixed()
let hours = hh === 1 ? `${hh} hour` : `${hh} hours`;
let minutes = mm === 1 ? `${mm} minute` : `${mm} minutes`;
document.getElementById('result').textContent = `${hours} ${minutes}`
}
The function passes the hours and minutes variable values into the textContent of the paragraph tag in the HTML.
Try this little hours and minutes app yourself by typing a number into the input field below.
There are of course excellent APIS to help with complexities of date and time. developers. See such resources on my JavaScript dev resources page.
That’s it.
Thank you for stumbling across this website and for reading my blog post entitled Calculate and display hours and minutes with JavaScript