- #javaScript
- #arrays
- #snippets
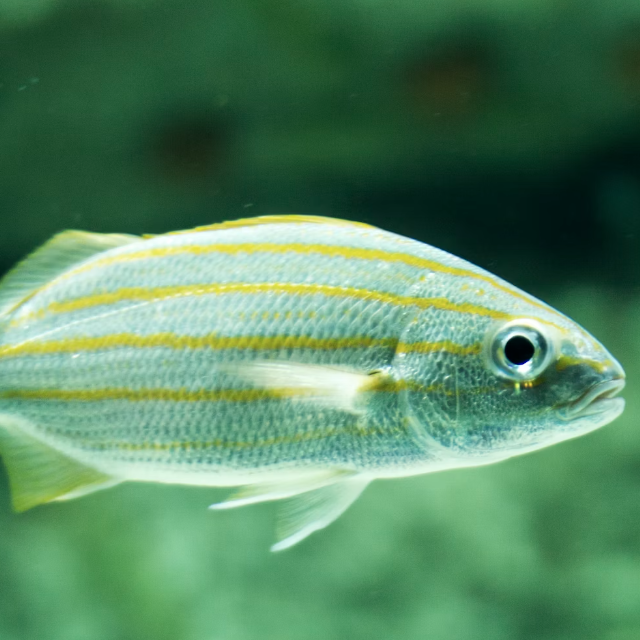
Introduction
There comes a time when you are working with arrays and you need to remove matching elements, or duplicates from the array.
Using the Set() object to remove duplicates
It is really worth playing around in the console dev tools to see what works and what doesn’t. Using numbers first, let’s see what does not work. Here is a nums array with a bunch of duplicated numbers.
const nums = [1, 3, 5, 7, 9, 2, 4, 1, 3, 7, 1, 2, 4, 6]
Consider the array nums. Obvs there are duplicates otherwise this demo would be pointless.
We now know there is a Set object. The solution must be as simple as passing in the array variable.
const firstTry = Set(nums)
❌ // Uncaught TypeError: Constructor Set requires 'new'
That didn’t work. Let’s try with new as suggested.
const secondTry = new Set(nums)
❌ // {1, 3, 5, 7, 9, …}
That didn’t work either. It returned an object-like but not an array. WTF?
const thirdTry = new Set([nums])
❌ // [[1, 3, 5, 7, 9, 2, 4, 1, 3, 7, 1, 2, 4, 6]
Oh FFS, that didn’t work either. It created a nested array and did not remove the duplicates.
const fourthTry = [new Set(nums)]
❌ // [{1, 3, 5, 7, 9, …}]
Oh FFS 😫. Close but no cigar.
const eureka = [...new Set(nums)]
✅ // [1, 3, 5, 7, 9, 2, 4, 6]
At last. So we figured the solution but does it work with string elements as well as numbers?
const names = ['Mike', 'Tin', 'Andy', 'Claire', 'Andy', 'Mike']
const unique_names = [...new Set(names)]
✅ // ['Mike', 'Tin', 'Andy', 'Claire']
Yaay!
There are other ways to remove duplicate elements from array but I think the Set() way is the cleanest. See my post Remove duplicate elements from array with loops for details.
Thank you for stumbling across this website and for reading my blog post entitled Remove duplicate elements from array with Set()