- #javaScript
- #arrays
- #snippets
- #objects
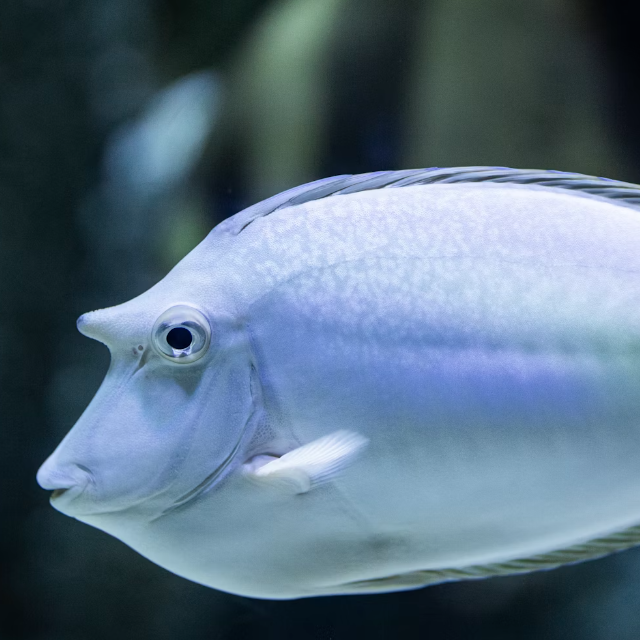
Removing an object from an array
In this example we have an array of things to do. Each object within the array has key value pairs including a ’complete: boolean’. The value would be true or false.
const things_to_do = [
{ task: 'Walk dog', complete: false, id: 0 },
{ task: 'Learn JavaScript', complete: false, id: 1 },
{ task: 'Get out of bed', complete: true, id: 2 },
];
Filter method
Now we can apply the filter() method to this things_to_do array to filter the things that are incomplete. Here a variable called ‘not_done_yet’ is created. Notice how the .filter() method is applied, its callback function looks for elements (el) that are false.
const not_done_yet = things_to_do.filter((el) => el.complete === false);
The not-yet-done variable returns:
[
{ task: 'Walk dog', complete: false, id: 0 },
{ task: 'Learn JavaScript', complete: false, id: 1 }
]
Using this technique, we could also filter the original array for things that have been completed. All we need to do is add true instead of false.
const done = things_to_do.filter((el) => el.complete === true);
Or indeed could we check for not false instead by using the exclamation mark !==
const done = things_to_do.filter((el) => el.complete !== false);
That’s it.
Thank you for stumbling across this website and for reading my blog post entitled Remove an object element from an array.