- #javaScript
- #snippets
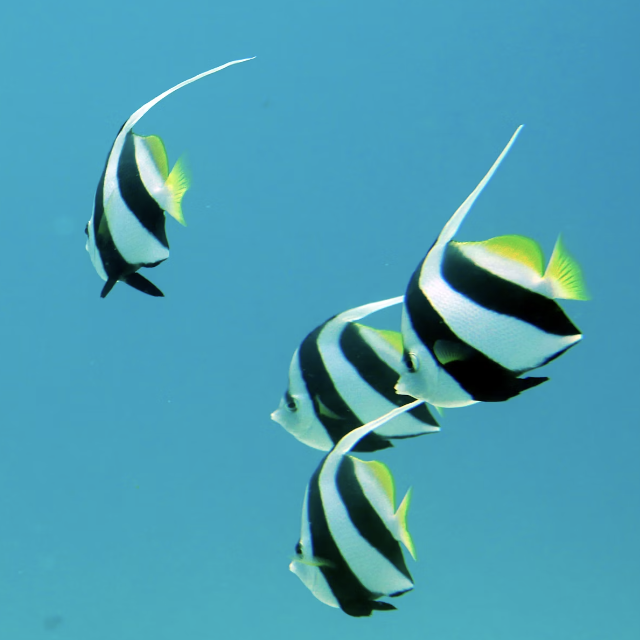
Introduction
I was developing an application that calculates the time difference between travelling a given distance at two different speeds. I wanted the answer in ’x minutes and y seconds’.
Time, distance, speed
The calculation of time is straightfoward. Distance divided by speed results in the time. But the resulting answer is in decimal minutes. For example, the time to travel 60 miles at 56 miles an hour is 64.29 minutes.
The problem to solve
From the example above the .29 is required to calculate seconds by multiplying it by 60. Noting that there are 60 seconds in a minute. There will be other ways to solve the problem but his is how I did it.
let number = 64.29
// 64.29
let decimal = Math.floor(number)
// 64
decimal = decimal - number
// .29
Of course we could create a reusable function to use on other integers, like this
function getDecimals(num) {
let decimal = Math.floor(num)
return decimal = num - decimal
}
getDecimals(number)
// 0.29000000000000004
It is typical of JavaScipt to go mad with the decimal places, so we could adjust our code with toFixed().
function getDecimals(num) {
let decimal = Math.floor(num)
return decimal = (num - decimal).toFixed(2)
}
getDecimals(number)
// '0.29'
But notice now a string is returned, therefore add a +.
function getDecimals(num) {
let decimal = Math.floor(num)
return decimal = +(num - decimal).toFixed(2)
}
getDecimals(number)
// 0.29
Now were have split the decimal from the number, we can use it for other calculations such as decimal minute to seconds.
let timeTaken = 64.29
let minutes = Math.floor(timeTaken)
// 64
let seconds = Math.floor(getDecimals(timeTaken) * 60)
// 17
<p>The time to cover the distance is `${minutes}` minutes and `${seconds} seconds.</p>
That’s it! I hope this helped 🚀.
Thank you for stumbling across this website and for reading my blog post entitled Split the decimal from a number in JavaScript