- #strings
- #javaScript
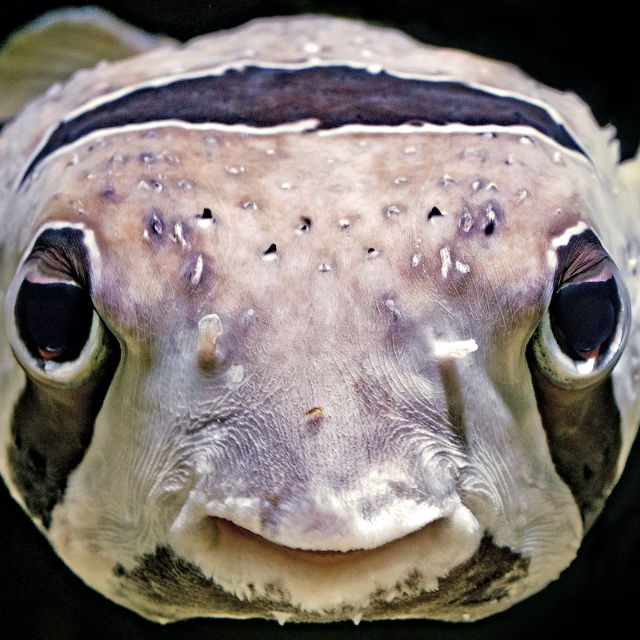
A to Z of string methods
at | padEnd | startsWith |
charAt | padStart | substring |
charCodeAt | repeat | toLowerCase |
codePointAt | replace | toUpperCase |
endsWith | replaceAll | trim |
indexOf | slice | trimEnd |
lastIndexOf | split | trimStart |
length |
String object
A string object represents a sequence of characters that can be manipulated.
const name = 'Michael';
const name_2 = new String('Michael');
.at()
To get the character at a postion in the string (zero based counting) or get the character at a reverse postion in the string (not zero based).
const name = 'Michael';
name.at(3); // returns h
name.at(-2); // returns e
.charAt()
To get the character at a postion in the string (zero based counting). This method does not work in the reverse sense.
const name = 'Michael';
name.charAt(3); // returns h
.charCodeAt()
To get the character code (UTF 16) at a postion in the string (zero based counting). This does not work in the reverse sense.
const name = 'Michael';
name.charCodeAt(4); // returns 97
For more information on UTF 16 characters, here is a useful web based Encoding Problem Table from ‘string functions’.
.charPointAt()
To get the code point value as a number (UTF 16) at a postion in the string.
const name = 'Michael';
name.codePointAt(3); // returns 104
.concat()
Join two or more strings together with concat.
const name = 'Mike';
name.concat(' ', 'is awesome'); // returns 'Mike is awesome'
.endsWith()
A boolean is returned.
const name = 'Michael';
name.endsWith('c'); // returns false
name.endsWith('l'); // returns true
.includes()
Does the string contain the case-sensitive character? A boolean is returned.
const name = 'Michael';
name.includes('m'); // returns false
name.includes('M'); // returns true
.indexOf()
Find the position of the first matching character. If there is no match -1 is returned.
const river = 'Mississippi';
river.indexOf('s'); // returns 2
river.indexOf('p'); // returns 8
river.lastIndexOf('w'); // returns -1
.lastIndexOf()
Returns the position of the last matching character. If there is no match -1 is returned.
const river = 'Mississippi';
river.lastIndexOf('s'); // returns 6
river.lastIndexOf('p'); // returns 9
river.lastIndexOf('w'); // returns -1
string length
Count the number of characters in a string with .length
const saying = 'I spy with my little eye';
saying.length; // returns 24
.padEnd()
Add ‘padding’ to the end of a string with a given number of characters.
const developer = 'My name is Mike';
developer.padEnd(20, '.'); // returns 'My name is Mike.....'
.padStart()
Adds ‘padding’ to the beginning of a string to a given number of characters.
const developer = 'My name is Mike';
developer.padStart(20, '.'); // returns '.....My name is Mike'
.repeat()
Return a new string which containing the specified number of copies of the orginal string.
const original = 'Happy birthday to you, ';
original.repeat(2); // returns 'Happy birthday to you, Happy birthday to you, '
.replace()
Replaces the first instance of the specified word.
const developer = 'My name is Mike. Mike is awesome';
developer.replace('Mike', 'Michael'); // returns 'My name is Michael. Mike is awesome'
.replaceAll()
Replaces the all instances of the specified word.
const dev = 'My name is Mike. Mike is awesome';
dev.replaceAll('Mike', 'Michael'); // returns 'My name is Michael. Michael is awesome'
.slice()
Extract characters from a string with slice.
const dev = 'My name is Mike. Mike is awesome';
dev.slice(-7); // returns 'awesome'
dev.slice(17); // returns 'Mike is awesome'
dev.slice(11, 21); // returns 'Mike. Mike'
.split()
Create an array of the string characters
const name = 'Michael';
name.split(); // returns ['Michael']
name.split(''); // returns ['M', 'i', 'c', 'h', 'a', 'e', 'l']
.startsWith()
A boolean returned on a case-sensitive match or otherwise of first character
const name = 'Michael';
name.startsWith('S'); // returns false
name.startsWith('M'); // returns true
.substring()
A new string is made from a selection of the original characters.
const dev = 'My name is Mike. Mike is awesome';
dev.substring(17); // returns 'Mike is awesome'
dev.substring(3, 10); // returns 'name is'
.toLowerCase()
Turns all characters to small case.
const name = 'Mike';
name.toLowerCase(); // returns 'mike'
.toUpperCase()
Turns characters to capitalised case.
const name = 'Mike';
name.toUpperCase(); // returns 'MIKE'
.trim()
Remove white space at both ends of the string.
const name = ' Mike ';
name.trim(); // returns 'Mike'
.trimEnd()
Remove white space at the end of the string.
const name = ' Mike ';
name.trimEnd(); // returns ' Mike'
.trimStart()
Remove white space at the beginning of the string.
const name = ' Mike ';
name.trimStart(); // returns 'Mike '
Thank you for stumbling across this website and for reading my blog post entitled String methods in JavaScript.