- #javaScript
- #arrays
- #snippets
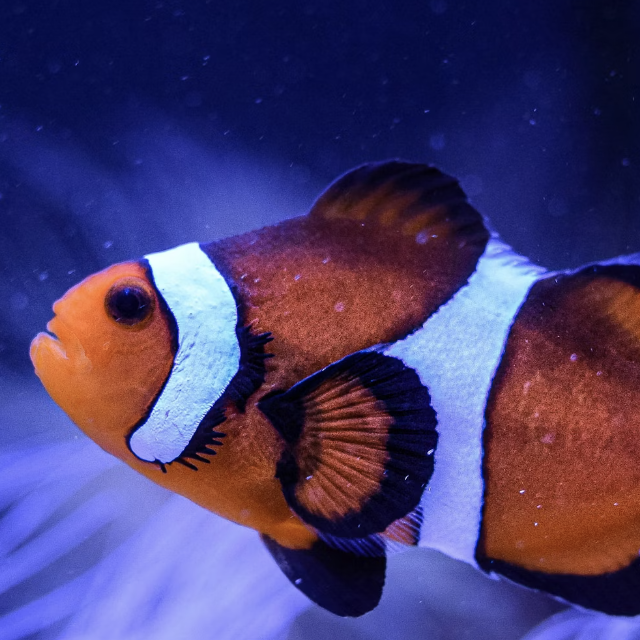
Create array of numbers
When declaring variables it is recommended that the variable name reflects what it is used for. Let’s create an array of numbers.
const numbersTosAdd = [10, 20, 30, 40, 50, 60, 70];
Sum of array with the .reduce() method
Now we can apply the reduce() method to the numbersToAdd array
const totalled = numbersTosAdd.reduce((totaller, nextNumberInArray) =>
totaller + nextNumberInArray, 0;
)
What is going on?
The function uses 2 parameters. To explain what is happening I named the parameters totaller and nextNumberInArray
totaller + nextNumberInArray, 0
The totaller starts with a value of 0. It adds the first number in the array to itself, becoming 10 in this case. It then adds the next number in the array (20) to itself, becoming 30. This is repeated until the last number in the array and the function stops.
Now log the variable totalled in the console.
The calculated total of the array in this instance is 280.
Snowball analogy
In his Udemy-based The complete Javascript course Jonas Schmedtmann usefully described my totaller like a snowball, rolling through the array getting bigger as it goes.
Sum of array with forEach()
Of course with JavaScript there are many ways of achieving the same thing. We can add up the array elements with the forEach() method.
First, create an array of numbers and a variable with the value of 0.
const arr1 = [1, 2, 3, 4, 5]
let sum1 = 0;
Loop the array adding each element to the sum1 variable
arr1.forEach(el => { sum1 += el })
console.log(sum1)
// 15
Sum of array with a for loop
Create an array of numbers and a variable with the value of 0.
const arr2 = [1, 2, 3, 4, 5]
let sum2 = 0;
Loop the array adding each element to the variable.
for (let i = 0; i < arr.length; i++) {
sum2 += arr2[i];
}
console.log(sum2)
// 15
Sum of array with a for of loop
Create an array of numbers and a variable with the value of 0.
const arr3 = [1, 2, 3, 4, 5]
let sum3 = 0;
Loop the array adding each element to the variable.
for (let i of arr3) { sum3 += i }
console.log(sum3)
// 15
References
Find more information about loops in my Loops in JavaScipt post.
Find more information about the Array.prototype.reduce() on MDN docs.
That’s it.
Thank you for stumbling across this website and for reading my blog post entitled Calculate the sum of array elements