- #snippets
- #javaScript
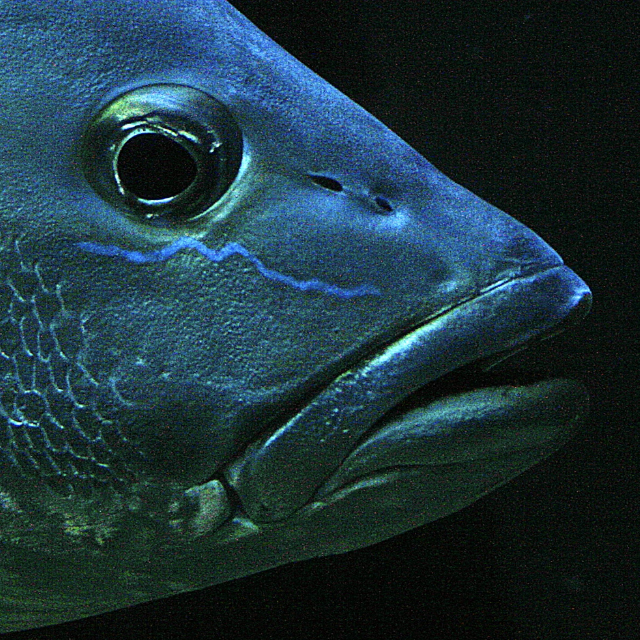
Introduction
Working with dates in JavaScript is not for the feint hearted. The key thing to remember is that an inbuilt Date object reflects the number of milliseconds since midnight on 1 January 1970 and that there are different methods that can be used on this figure. Let’s take a look.
Now
The now() method returns the current number of milliseconds since midnight on 1 January 1970.
const now = Date.now()
// 1706183315190
There are:
24 hours in a day
60 minutes in an hours
60 seconds in minute
1000 milliseconds in a second
Therefore there are:
(24 x 60 x 60 x 1000) milliseconds in a day.
(60 x 60 x 1000) milliseconds in a hour.
(60 x 1000) milliseconds in a minute.
Calculations can be made based on the Date.now() figure, for example using the value of the variable now the number of minutes since midnight on 1 January 1970 can be calculated.
`There have been ${now / (60 * 1000)} minutes since January 1, 1970`
`There have been ${now / (24 * 60 * 60 * 1000)} days since January 1, 1970`
That is enough about milliseconds!
Today
Get today’s date with the new Date() syntax. The returned date sytax can be seen below.
const today = new Date()
// Thu Jan 25 2024 13:16:35 GMT+0000 (Greenwich Mean Time)
That’s a lot to manipulate, so there are handy methods to make life a bit easier.
Year
At the bottom of this webage, I added a copyright notice with the year, which is dynamic and will change next year. Use the JavaScript .getFullYear() method to achieve this.
const thisYear = new Date().getFullYear();
// 2024
Date number
The .getDate() method returns the calendar number of the date.
const dateNum = new Date().getDate();
// 25
This date number might need to be portrayed as 25th or 25th. If so a conditional statement can be used to add ‘st’, ‘nd’, ‘rd’ or ‘th’ depending on the numeric value of the dateNum variable.
let num;
if (dateNum % 10 === 1) {
num = `${dateNum}${'st'}`;
} else if (dateNum % 10 === 2) {
num = `${dateNum}${'nd'}`;
} else if (dateNum % 10 === 3) {
num = `${dateNum}${'rd'}`;
} else {
num = `${dateNum}${'th'}`;
}
console.log(num)
// 25th
Month number
Similar to getting the date of the month, using the .getMonth() method returns the month itself. However, a zero-based count is returned, ie. 0 for the first month and 11 for the last month. We can maniplulate this figure with either conditional if or switch statements or array manipluation could be easier.
let x = new Date().getMonth()
const shortMonth = ['Jan', 'Feb', 'Mar', 'Apr', 'May', 'Jun', 'Jul', 'Aug', 'Sep', 'Oct', 'Nov', 'Dec']
const longMonth = ['January', 'February', 'March', 'April', 'May', 'June', 'July', 'August', 'September', 'October', 'November', 'December']
console.log(shortMonth[x], longMonth[x])
// Jan January
Hopefully by now you can see a trend in Date methods with the getDate(), getMonth() examples.
Day number
You may have guessed that the .getDay() method is used to return the day of the week. This time a zero-based figure is returned with 0 representing Sunday and 6 representing Saturday. Again, we can manipulate the returned value with arrays, like so:
let day = new Date().getDay();
const shortDay = ['Sun', 'Mon', 'Tue', 'Wed', 'Thu', 'Fri', 'Sat'];
const longDay = ['Sunday', 'Monday', 'Tueday', 'Wednesday', 'Thursday', 'Friday', 'Saturday'];
console.log(day, shortDay[day], longDay[day]);
// 4 Thu Thursday
console.log(`Tomorrow is ${longDay[day + 1]}`);
// Tomorrow is Friday
Calculate number of days until or days since
Having seen the logic with Date methods above, calculating the days between two dates should be relatively straightforward.
The following example calculates the number of days from, or since, today. The function takes in a day argument which must be a string, e.g. ‘1/23/2025’, or ‘2025-01-23’. The x variable is that argument converted to milliseconds since 1 Jan 1970. The y variable is the number of milliseconds at the time the function was called.
function daysFromToday(day) {
const x = new Date(day).getTime();
const y = Date.now();
const milliDay = 24 * 60 * 60 * 1000;
return Math.round((x - y) / milliDay);
}
Now that the x and y variables share common units they can be compared. Take one from the other and then divide by the number of milliseconds in a day to produce the number of days between the dates. A positive result means days until and a negative result reflects days since.
console.log(`The number of days between now and the end of the year is ${daysBetween('1/1/2025')}`)
// The number of days between now and the end of the year is 341
Calculate number of days between dates
The function above can be tweaked to take in two date arguments and calculate the number of days between them.
function daysBetweenDates(date_1, date_2) {
const x = new Date(date_1).getTime();
const y = new Date(date_2).getTime();
const milliDay = 24 * 60 * 60 * 1000;
return Math.round((x - y) / milliDay);
}
Do not forget that the date arguments must be strings and the arguments must follow the either syntax:
- ‘MM/DD/YYYY’.
- ‘YYYY-MM-DD’.
For example daysBetweenDates(‘2/14/2024’, ‘1/25/2024’), otherwise an error will occur.
Calculate number of weeks between dates
A similar approach can be used to calculate weeks between two dates. The new calculation for
function weeksBetweenDates(date_1, date_2) {
const x = new Date(date_1).getTime();
const y = new Date(date_2).getTime();
const milliWeek = 7 * 24 * 60 * 60 * 1000;
return Math.round((x - y) / milliWeek);
}
That’s it.
Thank you for stumbling across this website and for reading my blog post entitled Working with dates in vanilla JavaScript