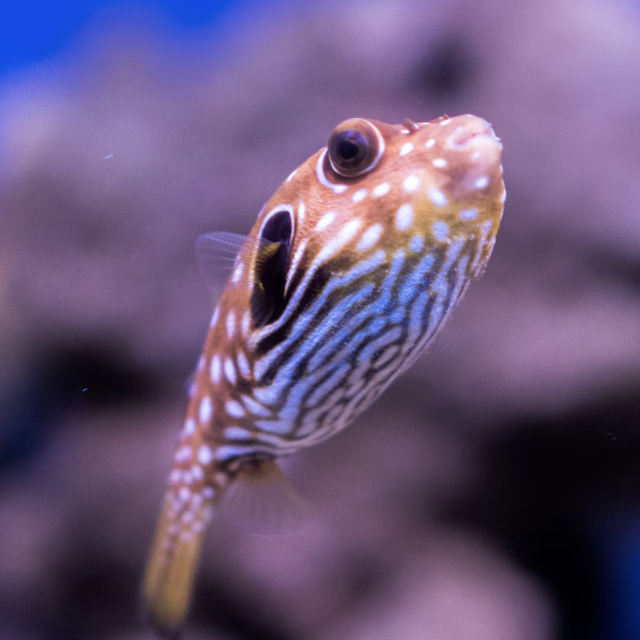
Coding Tetris with vanilla JavaScript
Thinking about the logic behind the Tetris game and associated JavaScript ideas
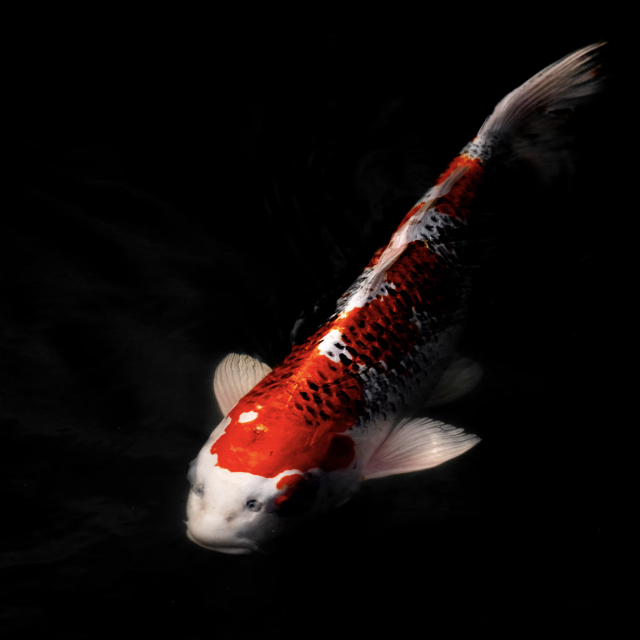
Array methods in JavaScript
Working examples of 34 JavaScript array methods.
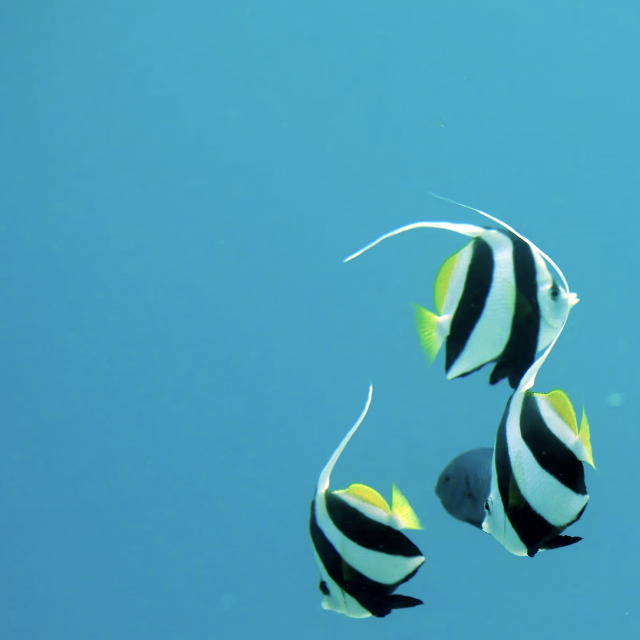
Check for matching values in two arrays with JavaScript
Learn how to find matching values between two arrays using JavaScript
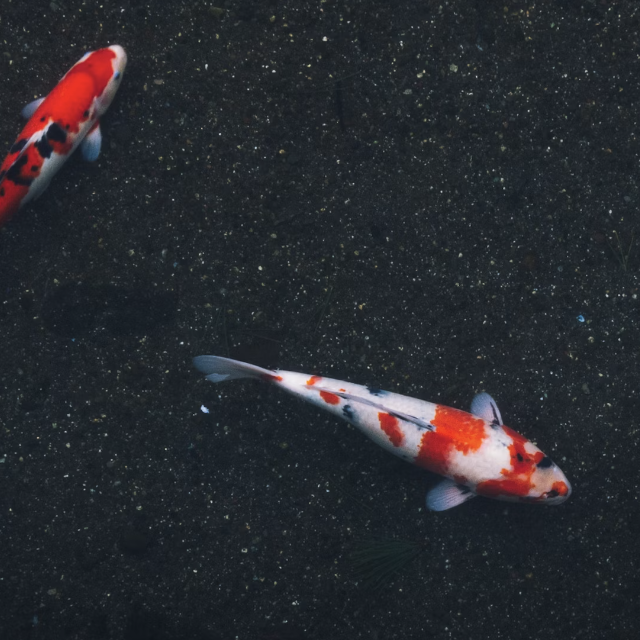
Dynamically shift HTML input focus to the next element
A guide on how to dynamically move the focus on HTML input elements if when first is filled in. This requires a little bit of JavaScript.
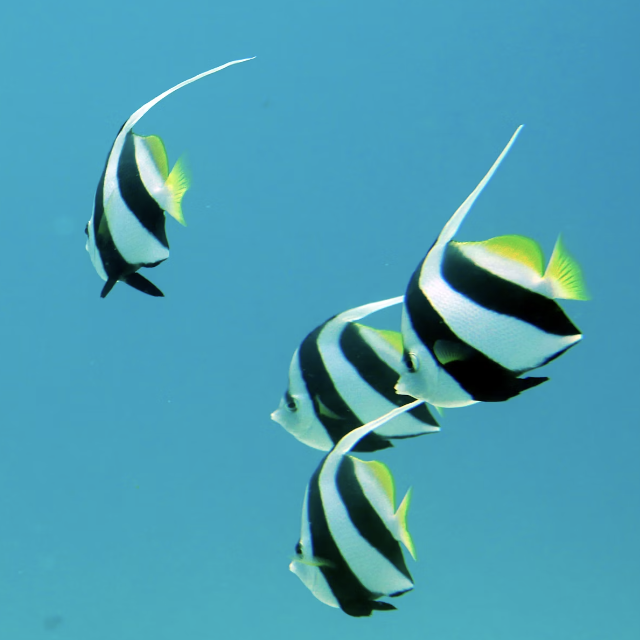
Split the decimal from a number in JavaScript
How to get the decimal element from an integer using JavaScript.
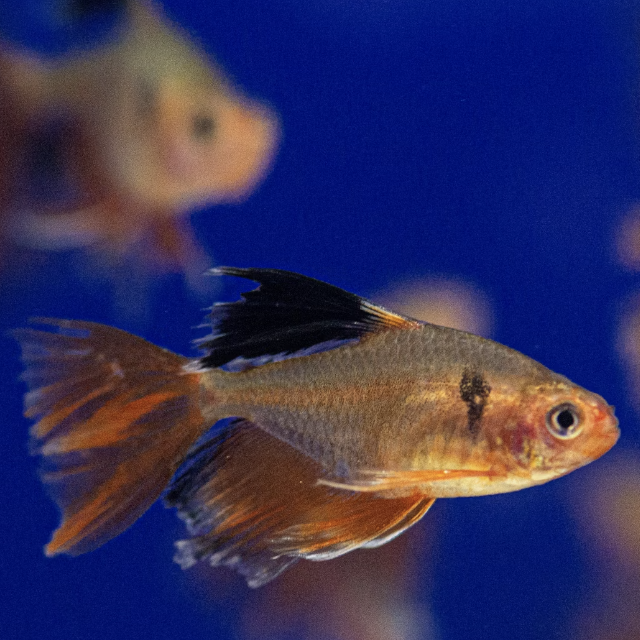
Making a CRUD app with Svelte 5
How I programmed a create, read, update and delete app with Svelte 5.
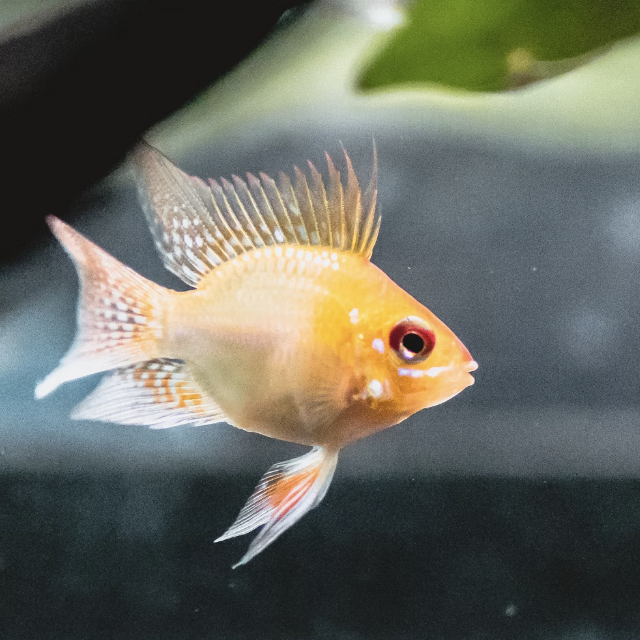
Shuffle array items with JavaScript
How to shuffle array items with JavaScript.
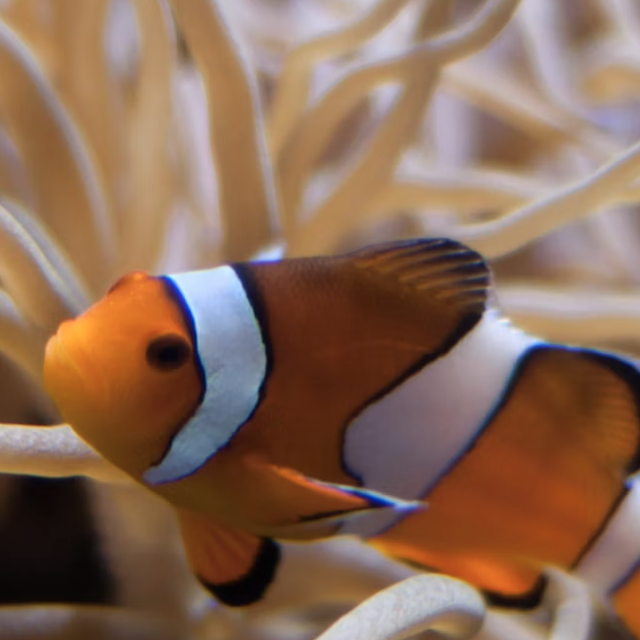
Round a decimal number down with JavaScript
How to round a decimal number down with JavaScript
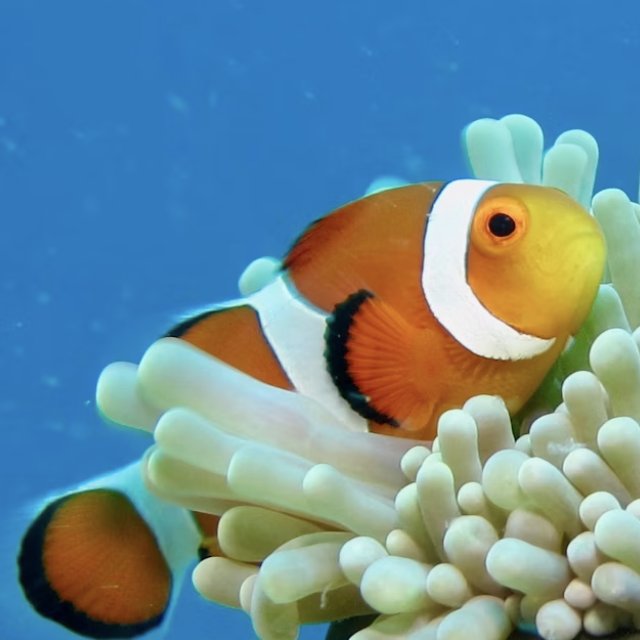
Round a decimal number up with JavaScript
How to round a decimal number up with JavaScript
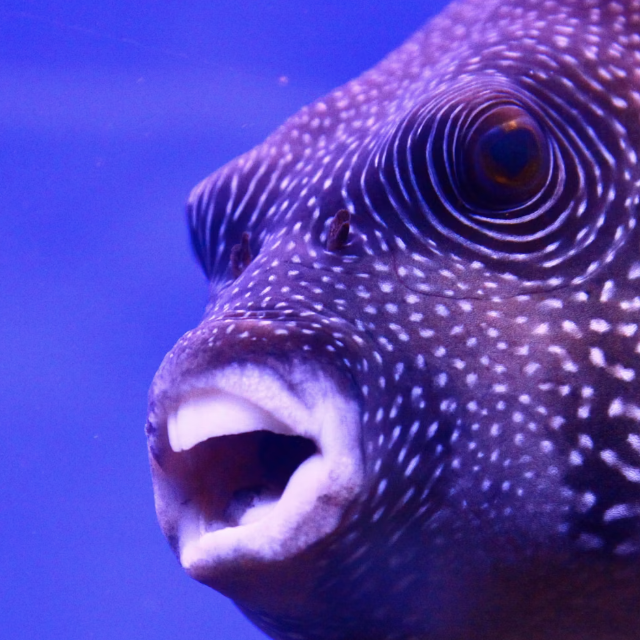
Arrays in JavaScript
A guide to understanding arrays and their use in JavaScript programming.
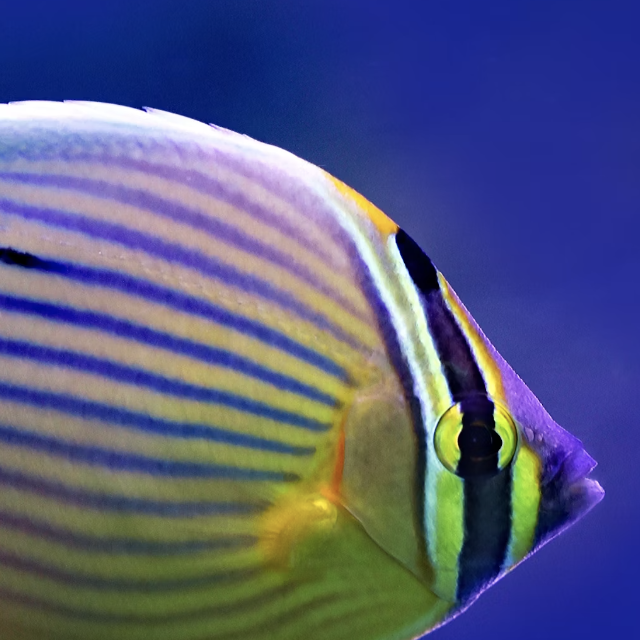
Rethinking 2048
Coding the 2048 game with vanilla JavaScript.
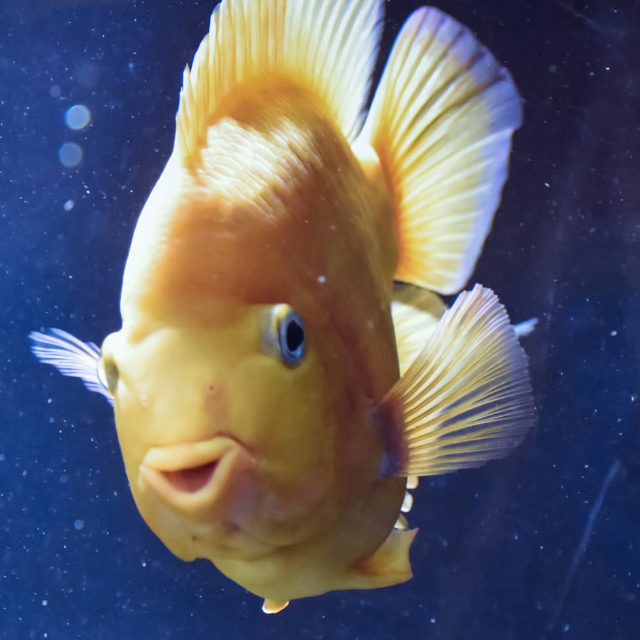
Add a tax percentage to array of prices
Ways to add a percentage to array elements.
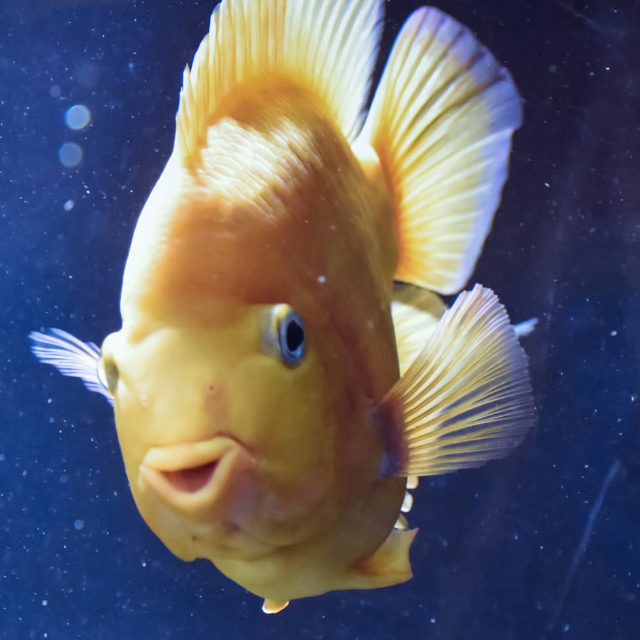
Find element in array and delete it
Find an array element and delete it with splice().
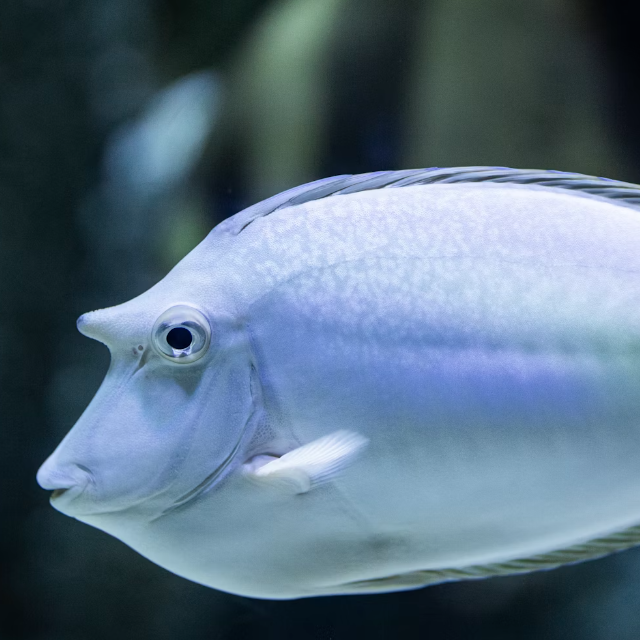
Remove an object element from an array
Removing an object element from an array is possible using the 'filter()' method.
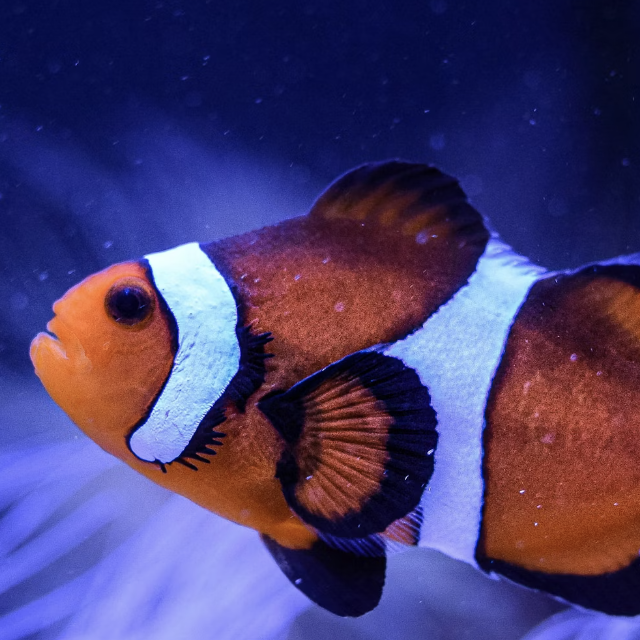
Calculate the sum of array elements
Calculate the sum of array elements with the reduce() method.
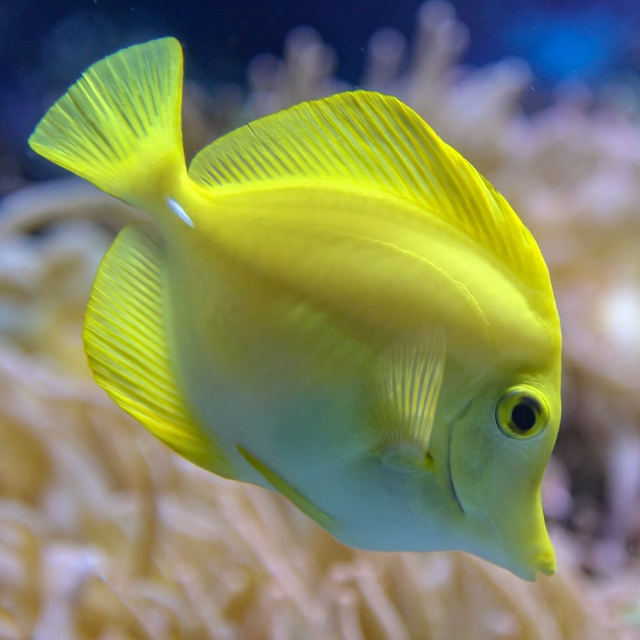
How to add elements to and remove elements from an array
Add elements to the beginning and end of an array with the unshift and push methods. Remove them using the pop and shift methods.
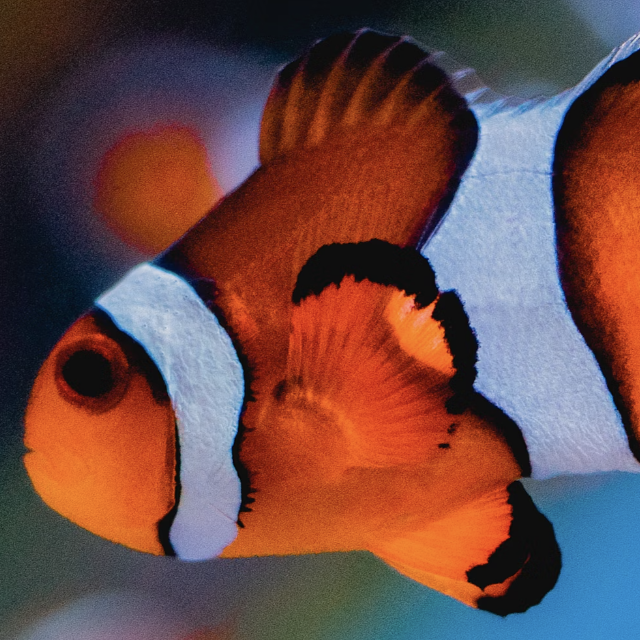
Remove duplicate elements from array with loops
Removing duplicate elements from array with loops and the includes() method.
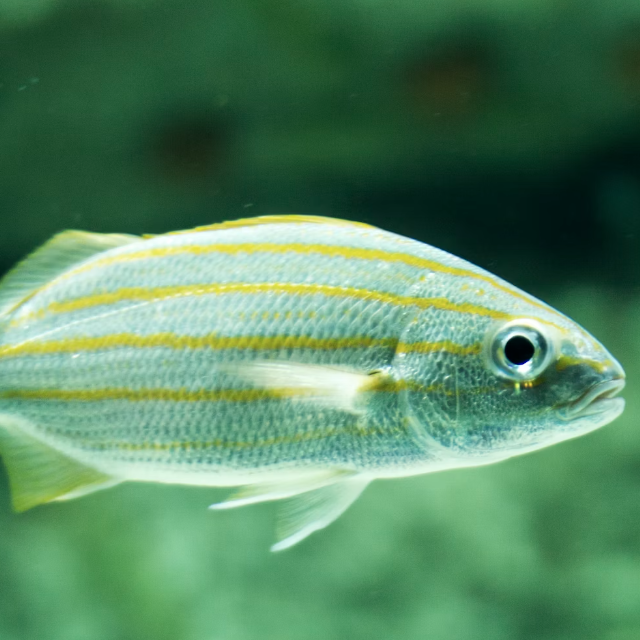
Remove duplicate elements from array with Set()
Removing matching array elements is easy with Set().
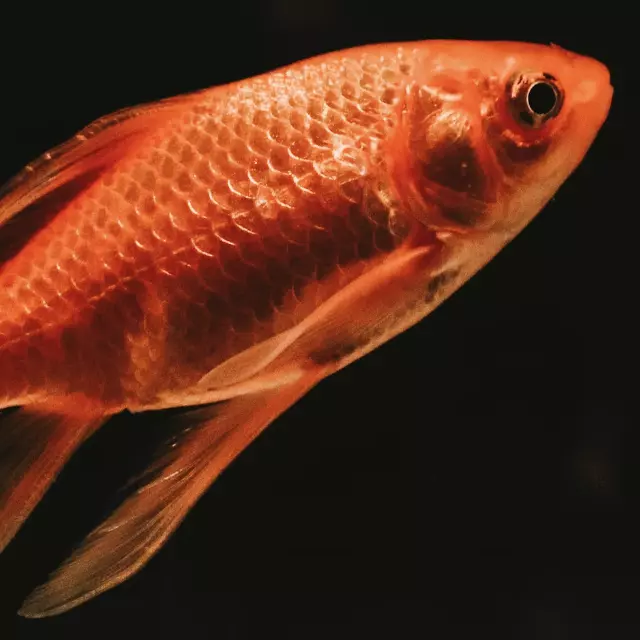
Notes on DOM manipulation
Elements on a website can be controlled and manipulated with JavaScript. This post looks at how this can be achieved.
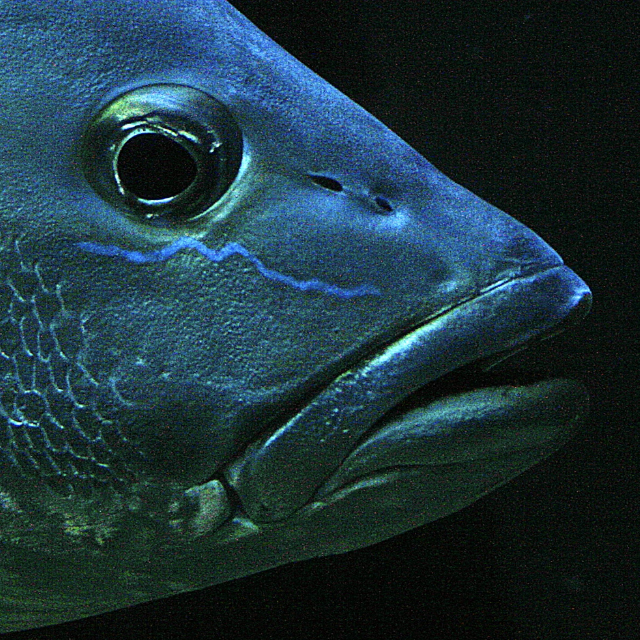
Working with dates in vanilla JavaScript
Working with dates in JavaScript including today, year, date number, month number and day number.
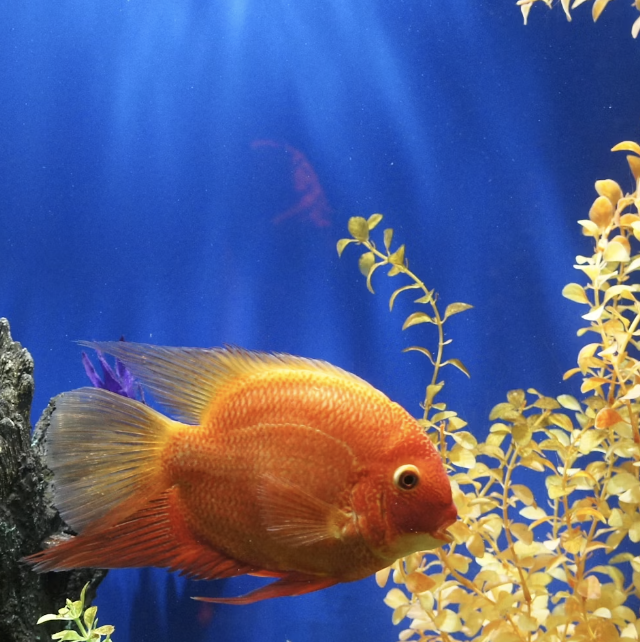
Loops in JavaScript
Looping, or iterating, over objects is essential in JavaScript programming.
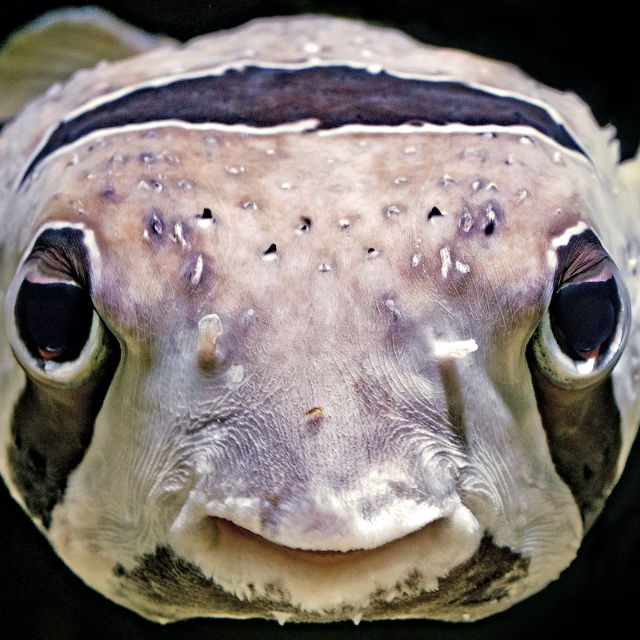
String methods in JavaScript
Examples of inbuilt string methods, or functions, that are used for manipulating string data.
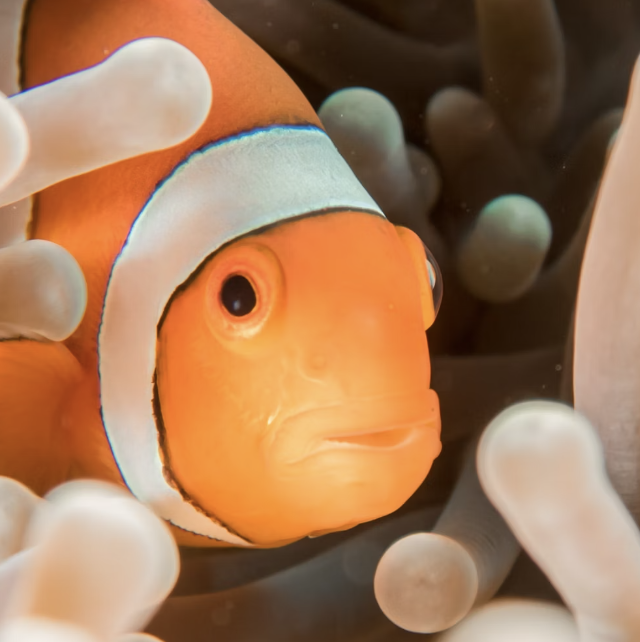
JS Arrays in numerical order
Sorting array elements in numerical order with Javascript.
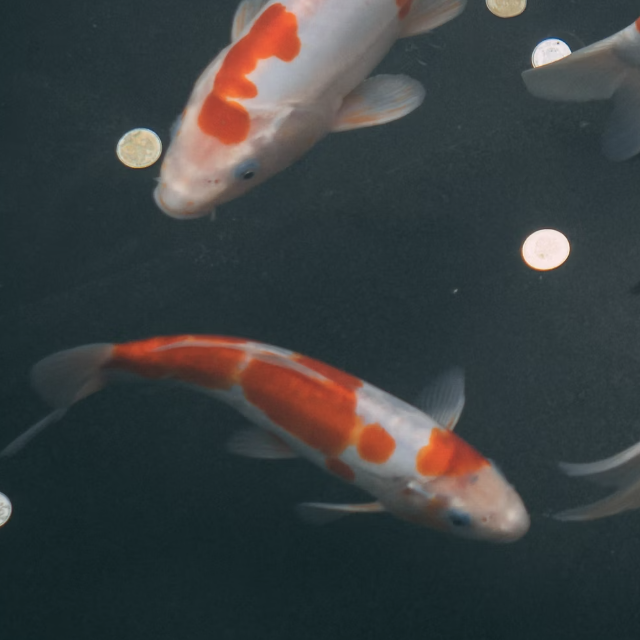
Calculate and display hours and minutes with JavaScript
This JavaScript snippet calculates and displays hours and minutes from a user's number input.
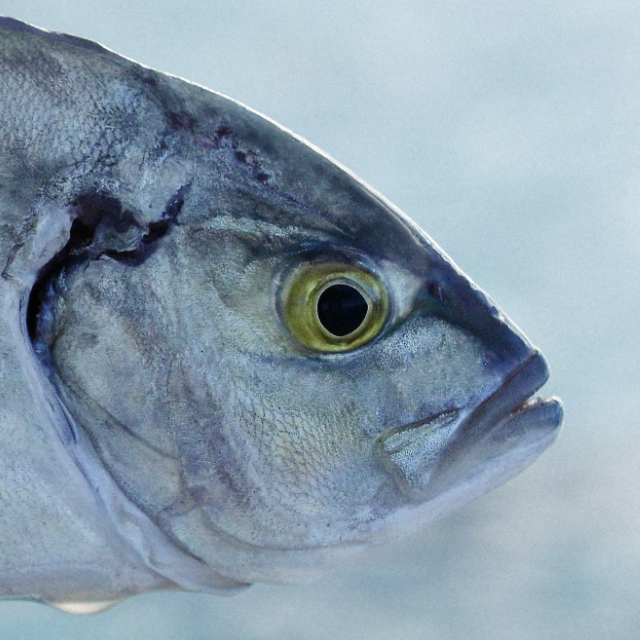
CSS and JavaScript for website dark mode
How to create a dark mode on a website
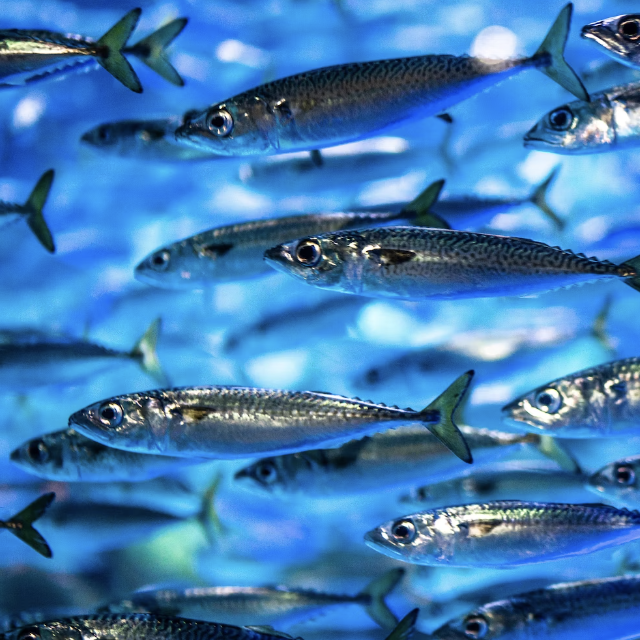
JavaScript resources for developers
List of handy JavaScript related resources for developers.
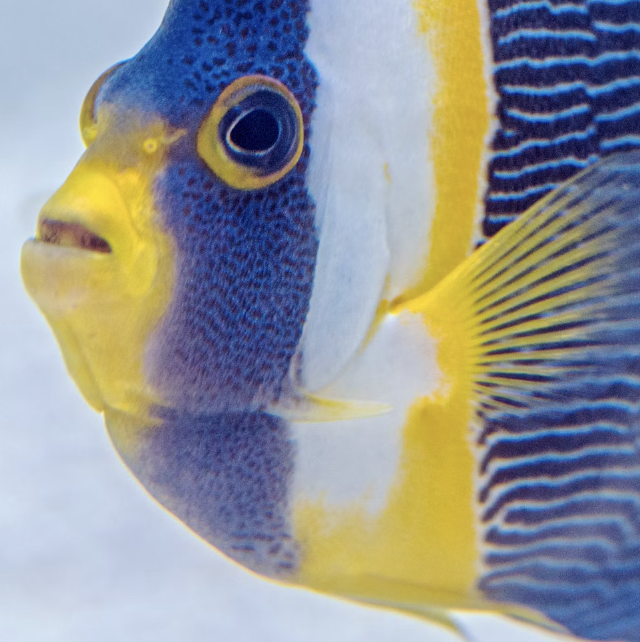
Objects in JavaScript
A guide to Objects and their use in JavaScript programming.